I discovered that PHP GD library can be very useful and powerful when it comes to creating and image that will be automatically generated from the supplied data.
Bar charts are very useful when it comes to presentation just like the popular saying "A picture is worth a thousand words." . Not only that it very useful to web developers when it comes to displaying analytic on the web applications. If you’re the type that loves to create your own design and have your own thing am sure you are going to love this. In this Tutorial we are going to use PHP GD library to create a bar chat and as for the demo it is going to be displaying the results of students for their exam, test and practical.
You can customize the source code to suit whatever you want to use it with the code is very flexible and easy to manipulate.
I am not using database to serve the data bus as a replacement an array was used which is the same data structure any database query result will use.
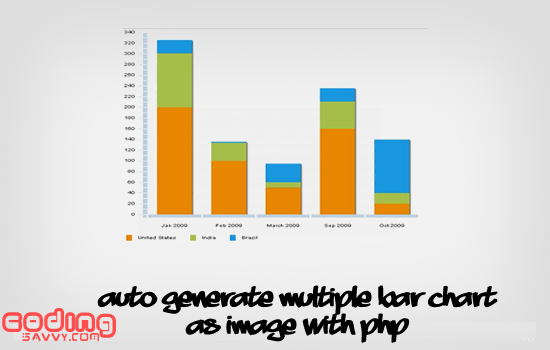

First let define width and height for our Canvas :
define('WIDTH', 300);
define('HEIGHT', 250);
define('HEIGHT', 250);
Now let create a demo array that will contain all courses and the results of the student
//Create a random data
$data = array("MEE212"=>(array("C.A"=>10,"exam"=>40,"Practical"=>25)),"ELE201"=>(array("C.A"=>22,"exam"=>30)),"ELE257"=>(array("C.A"=>22,"exam"=>30)),
"CHE212"=>(array("C.A"=>16,"exam"=>32,"Practical"=>15)),"CVE212"=>(array("C.A"=>11,"exam"=>20,"Practical"=>10)));
$data = array("MEE212"=>(array("C.A"=>10,"exam"=>40,"Practical"=>25)),"ELE201"=>(array("C.A"=>22,"exam"=>30)),"ELE257"=>(array("C.A"=>22,"exam"=>30)),
"CHE212"=>(array("C.A"=>16,"exam"=>32,"Practical"=>15)),"CVE212"=>(array("C.A"=>11,"exam"=>20,"Practical"=>10)));
Creating image with the specified width and height above :
// Create the image
$img = imagecreatetruecolor(WIDTH, HEIGHT);
$img = imagecreatetruecolor(WIDTH, HEIGHT);
Now to colourise the bars I use random color for some while some bar items have a specific color You can always use any color you like the color is in RGB fomart.:
// Set a white background with black text and gray graphics
$bg_color = imagecolorallocate($img, 255, 255, 255); // white
$text_color = imagecolorallocate($img, 0, 0, 0); // black
$graphic_color = imagecolorallocate($img, 64, 64, 64); //
$exam_color =imagecolorallocate($img, (int)rand(1,50), (int)rand(1,91), (int)rand(1,109)); //random color
$test_color = imagecolorallocate($img,217, 86, 69);
$score_color = imagecolorallocate($img,231, 231, 231);
Let create a rectangle and fill it with our background color
I f you note here the Image instance that was created earlier is part of our parameter because it is required by the function:$bg_color = imagecolorallocate($img, 255, 255, 255); // white
$text_color = imagecolorallocate($img, 0, 0, 0); // black
$graphic_color = imagecolorallocate($img, 64, 64, 64); //
$exam_color =imagecolorallocate($img, (int)rand(1,50), (int)rand(1,91), (int)rand(1,109)); //random color
$test_color = imagecolorallocate($img,217, 86, 69);
$score_color = imagecolorallocate($img,231, 231, 231);
imagefilledrectangle($img, 0, 0, WIDTH, HEIGHT, $bg_color);
Using the amount of data in the Array to calculate how long our Y and X axis will be taking the specified Chart width and height into consideration.
imageline($img,(count($data)*50),HEIGHT-18,30,HEIGHT-18,$text_color);//X
imageline($img, 30, 18, 30, HEIGHT-20, $text_color);//Y
Using PHP foreach loop let loop through our data and transform it into bars try to study this aspect gently because this is the most interesting part.
// Draw a bar
$r=1;
foreach ($data as $course=>$result):
$rectx1 = 35*$r;
$form=1;
$start =0;
$stop=0;
foreach ($result as $test => $score):
$exam_color =imagecolorallocate($img, (int)rand(1,50), (int)rand(1,91), (int)rand(1,109));
$strx =36*$r;
$strpt = 0.9;
$start = ($start==0)?HEIGHT-20:$stop;
$stop =($stop==0)? HEIGHT-20-(($score)*2): $start-($score*2);
imagefilledrectangle($img, $rectx1, $stop, $rectx1+20, $start, $exam_color);//exam
imagestringup($img, $strpt, $strx, $start-2, $score, $score_color);
$start++;
endforeach;
imagestring($img,0.4,36*$r,HEIGHT-15,$course,$text_color);
$r++;
endforeach;
$r=1;
foreach ($data as $course=>$result):
$rectx1 = 35*$r;
$form=1;
$start =0;
$stop=0;
foreach ($result as $test => $score):
$exam_color =imagecolorallocate($img, (int)rand(1,50), (int)rand(1,91), (int)rand(1,109));
$strx =36*$r;
$strpt = 0.9;
$start = ($start==0)?HEIGHT-20:$stop;
$stop =($stop==0)? HEIGHT-20-(($score)*2): $start-($score*2);
imagefilledrectangle($img, $rectx1, $stop, $rectx1+20, $start, $exam_color);//exam
imagestringup($img, $strpt, $strx, $start-2, $score, $score_color);
$start++;
endforeach;
imagestring($img,0.4,36*$r,HEIGHT-15,$course,$text_color);
$r++;
endforeach;
The first Line is creating a vertical string for the axis label for the Y axis while the second line ( for loop) Is creating label for each units on the Y axis remember in our previous looping through the data we had already created a label for the X-axis.
imagestringup($img,10, 3, 150,"scores",$text_color);
for ($i=0;$i<11;$i++){
imagestringup($img,0.2, 20, 230-($i*20),$i*10,$text_color);
}
Finally Let output the image (line 1-2) and delete the junks(line 3) that has been created on the server.
header("Content-type: image/png");
imagepng($img);
imagedestroy($img);
for ($i=0;$i<11;$i++){
imagestringup($img,0.2, 20, 230-($i*20),$i*10,$text_color);
}
Finally Let output the image (line 1-2) and delete the junks(line 3) that has been created on the server.
header("Content-type: image/png");
imagepng($img);
imagedestroy($img);
Thanks!
Your feedback helps us improve tutorials.
No comments:
Post a Comment